PHP String – How to Create a String and Use String Functions
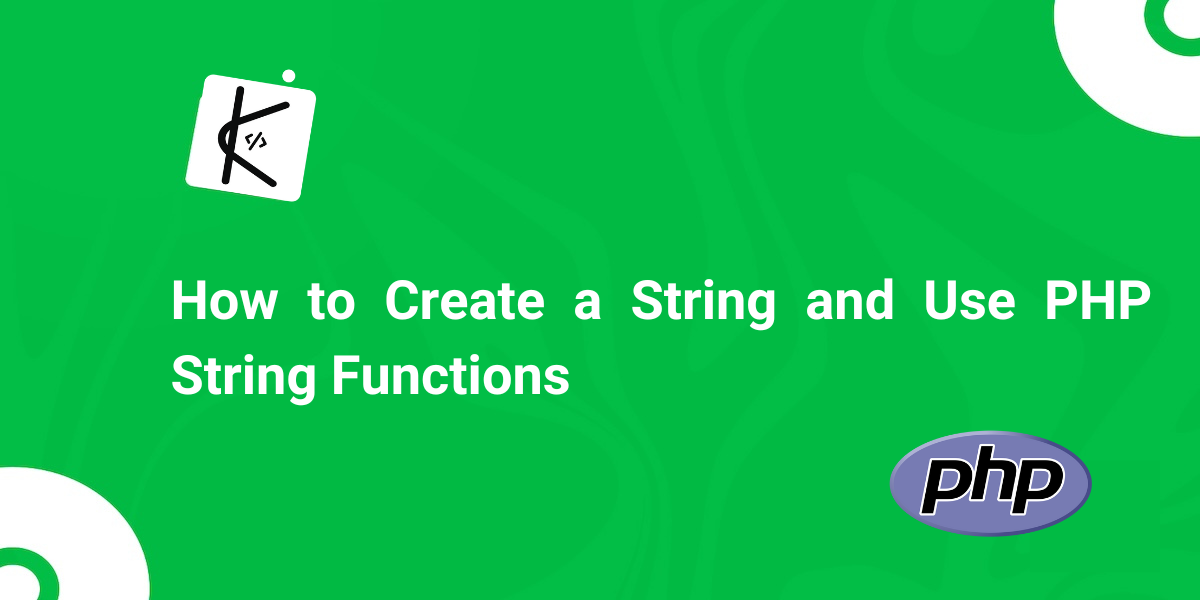
Written by Kolade Chris | Jul 14, 2024 | #PHP | 8 minute Read
String is one of the fundamental data types in PHP and other programming languages. It is used extensively in web development for representing textual data.
In PHP, a string is a sequence of characters, such as letters, numbers, symbols, or whitespace, enclosed within either single quotes (''
) or double quotes (""
).
How to Create a String in PHP
The commonest way to create a string is to surround the characters with single or double quotes:
1$singleQuoteString = 'John Doe';2$doubleQuoteString = "Jane Doe";
Apart from surrounding a sequence of characters with single or double quotes to make it a string, there are other ways you can create a string in PHP. There are heredoc
and nowdoc
strings which allow you to create strings that span multiple lines.
Heredoc
string behaves like a string surrounded by double quotes, and nowdoc
string behaves like a string created with single quotes.
To create a heredoc
string, you start with triple lesser than symbols (<<<
), then the identifier for the variable, and end with that same identifier:
1$myHereDocString = <<<EOD2This is a heredoc string3It lets you create variables that4span multiple lines5EOD;
If you want to create a nowdoc
string, you start with triple lesser than symbols followed by the identifier too, but you have to surround the identifier with a single quote:
1$myNowdocString = <<<'NOD'2This is a nowdoc string3It also lets you create strings4that span multiple lines5but works like a string with single quotes6NOD;
How to Concatenate Strings in PHP
Concatenation is the process of combining two or more strings. There are several ways to concatenate variables in PHP. These include:
- using the concatenation operator, which is a dot (
.
) in PHP - compound concatenation assignment
- curly [syntax] braces
- and using the implode function with arrays
How to Concatenate Strings with the Concatenation Operator
You can concatenate two or more strings by putting a dot (.
) between them:
1$firstname = 'John';2$lastName = 'Doe';3
4$fullName = $firstname . ' ' . $lastName;5echo $fullName // John Doe
You can also concatenate more than two strings more explicitly:
1$firstname = 'John';2$lastName = 'Doe';3$middleName = 'Chris';4
5$fullName = $firstname . ' ' . $middleName . ' ' . $lastName;6echo $fullName // John Chris Doe
How to Concatenate Strings with the Compound Concatenation Assignment Operator
The compound concatenation assignment operator is represented by .=
. This operator appends the right operand to the left operand:
1$greeting = "Hello";2$name = "John";3
4$greeting .= " " . $name;5echo $greeting; // Hello John
How to Concatenate Strings By Using Double Quotes
PHP allows you to interpolate strings and other variables directly inside a string using double quotes:
1$sport = 'Football';2$bestSport = "My best sport is $sport";3
4echo $bestSport; // My best sport is Football
Since a heredoc
string behaves like a string created with double quotes, it means you can do the same thing with it:
1$myStr = "Hello world";2$myHereDocString = <<<EOD3$myStr from a heredoc string4Remember this type of string5lets you create strings spanning6mutiple lines7EOD;8
9echo $myHereDocString;10/* Hello world from a heredoc string11Remember this type of string12lets you create strings spanning13mutiple lines */
How to Concatenate Strings By Using Curly Braces
You can use curly braces inside a string with double quotes to concatenate two or more variables. Here’s an example:
1$firstName = 'John';2$lastName = 'Doe';3$middleName = 'Chris';4
5$fullName = "My full name is {$firstName} {$middleName} {$lastName}";6
7echo $fullName; // My full name is John Chris Doe
How to Concatenate Strings With the implode()
Function
implode()
is an array method you can use to combine the elements of an array of strings into one string.
implode()
takes two parameters – a delimiter and the array to use. The delimiter is what will separate each element of the array.
1$words = array("Hello", "world!");2
3echo implode(" ", $words); // Hello world!
Here’s an example using an array created with square brackets:
1$colors = ["red", "green", "blue"];2$commaSeparatedColors = implode(", ", $colors);3
4echo $commaSeparatedColors; // red, green, blue
Join my Free Newsletter
Subscribe to my newsletter for coding tips, videos from reputable sources, articles from OG tech authors, and a ton of other goodies.
No BS. No fluff. Just pure software development goodies on a Sunday every week.
String Functions in PHP
PHP provides a variety of built-in string functions that allow you to manipulate, search, format, and extract information from strings.
Below are some commonly used string functions in PHP:
The strlen()
Function
The strlen()
function returns the length of the characters in a string
1$myStr = "PHP is awesome!";2
3echo strlen($myStr); // 15
The str_word_count()
Function
This function takes a string as the parameter and returns the length of the words in that string:
1$myStr = "Hello world, PHP is awesome!";2
3echo str_word_count($myStr); // 5
The substr()
Function
The substr() function in PHP is used to extract a substring from a larger string. It takes three parameters:
String
: The original string from which you want to extract the substring.Start
: An integer, it’s the starting position from where the substring extraction begins. If it’s positive, counting starts from the beginning of the string. If it’s negative, counting starts from the end of the string.Length
(optional): Also an integer, it’s the length of the substring to extract. If omitted,substr()
extracts everything from the starting position to the end of the string.
The general syntax looks like this:
1substr(string, start, length):
You can extract a substring from a specific position:
1$myStr = "Hello, world!";2$substring = substr($myStr, 7);3
4echo $substring; // world!
You can specify the optional length to indicate how many characters you want:
1// extract world without the exclamation mark2$myStr = "Hello, world!";3$substring = substr($myStr, 7, 5);4
5echo $substring; // world
You can use negative starting position to extract from the end of the string:
1$myStr = "Hello, world!";2$substring = substr($myStr, -6);3
4echo $substring; // world!
You can also specify a length after using a negative starting index:
1$myStr = "Hello, world!";2$substring = substr($myStr, -13, 5);3
4echo $substring; // Hello
The strpos()
Function
strpos()
returns the position of the first occurrence of a substring within a string.
1$myStr = "Hello, world!";2
3echo strpos($myStr, "!"); // 12
The strtoupper()
Function
strtoupper()
takes a string and converts it to uppercase:
1$myStr = "Hello, world!";2
3echo strtoupper($myStr); // HELLO, WORLD!
The strtolower()
Function
strtolower()
is the opposite of strtoupper()
. It takes a string and converts it to lowercase:
1$myStr = "Hello, world!";2
3echo strtolower($myStr); // hello, world!
The str_replace()
Function
The str_replace()
function takes three compulsory parameters:
- the target string to replace
- the replacer string
- the whole string containing the target string – this could be a variable.
1$myStr = "Hello, world!";2
3echo str_replace("Hello", "Hi", $myStr) . '<br>'; // Hi, world!4echo str_replace("world!", "planet earth!", $myStr); // Hello, planet earth!
The ucwords()
Function
The ucwords()
function takes a string and makes the first letter of each word in it uppercase. In some languages, this is not built-in at all!
1$myStr = "hello, world!";2
3echo ucwords($myStr); // Hello, World!
Wrapping Up
Mastering strings in PHP is essential for building dynamic websites. Using different methods like single quotes or double quotes, and also heredoc
and nowdoc
, you can create strings flexibly.
As this article discusses, PHP offers valuable functions like strlen()
or substr()
for tasks like finding string length or extracting substrings, simplifying the development process. With those functions, you can efficiently handle textual data and build user-friendly applications that meet modern web development standards.