JavaScript Format Number as Currency – How to Format Currencies in JavaScript
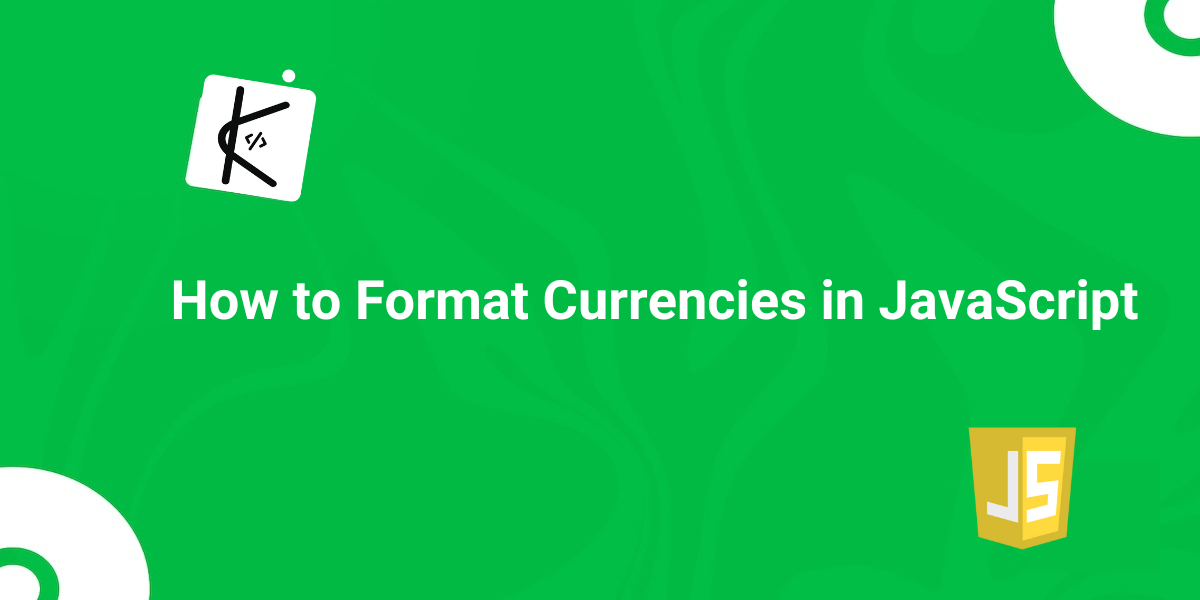
Written by Kolade Chris | Sep 28, 2024 | #JavaScript | 5 minutes Read
While working on an eCommerce website using JavaScript, financial reports, or dealing with product data from an API, you’ll need to format certain numbers or strings as currencies or convert them to currencies.
You’ve come to the right place if you’re looking into how to format numbers as currency strings in JavaScript.
In this article, I’ll show you the manual approach first, then proceed to show you how to do it with the JavaScript Internationalization API (Intl Constructor)
.
The Manual Approach: A Generic Function that Takes a Number or String and Converts it to Currency
You could create a function that takes a number or string and converts it to your target currency:
const formatCurrency = (amount) => { amount = parseFloat(amount);
if (isNaN(amount)) { return 'Invalid amount'; }
let formattedAmount = amount.toFixed(2).toString().split('.');
formattedAmount[0] = formattedAmount[0].replace(/\B(?=(\d{3})+(?!\d))/g, ',');
return '$' + formattedAmount.join('.');};
console.log(formatCurrency('902.4')); // $902.40console.log(formatCurrency(82.65)); // $82.65
This approach is not sustainable because it handles one currency.
What if you want to handle many other currencies? Well, you can modify the function to accept a currency symbol:
const formatCurrency = (amount, currencySymbol) => { amount = parseFloat(amount);
if (isNaN(amount)) { return 'Invalid amount'; }
let formattedAmount = amount.toFixed(2).toString().split('.');
formattedAmount[0] = formattedAmount[0].replace(/\B(?=(\d{3})+(?!\d))/g, ',');
return currencySymbol + formattedAmount.join('.');};
console.log(formatCurrency('902.4', '₦')); //₦902.40console.log(formatCurrency(82.65, '€')); // €82.65
This approach is still not sustainable because it requires custom logic for different locales and currencies, making it complex and error-prone.
There’s a solution, though. That solution is the Intl.NumberFormat
object of the JavaScript Internationalization API.
Pardon the interruption…
Join my Free Newsletter
Subscribe to my newsletter for coding tips, videos from reputable sources, articles from OG tech authors, and a ton of other goodies.
No BS. No fluff. Just pure software development goodies on a Sunday every week.
Now unto how to format currencies with the Intl.NumberFormat
object…
How to Format Numbers as Currency with the Intl.NumberFormat()
Constructor
Using the Intl.NumberFormat()
constructor to format numbers as currency is an improvement over manual methods. It streamlines the process by automatically applying locale-specific rules. It also offers built-in support for various currencies.
You can create an instance of the Intl.NumberFormat
with the new keyword or without it:
new Intl.NumberFormat(); // works fineIntl.NumberFormat(); // works fine
You can also assign it to a variable:
const formatCurrency = Intl.NumberFormat();
The Intl.NumberFormat()
constructor takes two parameters – locale
and options
. It uses the .format()
method to do the actual number formatting.
Both parameters are optional, so you can use the Intl.NumberFormat()
constructor directly. This way, it’ll only add commas to the numbers to indicate it in either thousand, million, or more:
const formatThousand = Intl.NumberFormat().format(98932);console.log(formatThousand); // 98,932
const formatMillion = Intl.NumberFormat().format(48921043);console.log(formatMillion); // 48,921,043
If you decide to use only the locale parameter, the number will be formatted as done in that location. Here’s what that looks like for Nigeria, Italy, and UK:
const amount = 369741564;
// Formatting for Nigeriaconsole.log(new Intl.NumberFormat('en-NG').format(amount)); // 369,741,564
// Formatting for Italyconsole.log(new Intl.NumberFormat('it-EU').format(amount)); // 369.741.564
// Formatting for the United Kingdomconsole.log(new Intl.NumberFormat('en-GB').format(amount)); // 369,741,564
If you finally use the options
parameter with style
set to 'currency'
and currency
set to the currency in that locale, the number will be formatted as currency:
const amount = 369741564;
// Formatting currency for Nigeriaconsole.log( new Intl.NumberFormat('en-NG', { style: 'currency', currency: 'NGN' }).format( amount )); // ₦369,741,564.00
// Formatting currency for Italyconsole.log( new Intl.NumberFormat('it-EU', { style: 'currency', currency: 'EUR' }).format( amount )); // 369.741.564,00 €
// Formatting currency for the United Kingdomconsole.log( new Intl.NumberFormat('en-GB', { style: 'currency', currency: 'GBP' }).format( amount )); // £369,741,564.00
Finally, you can create a reusable function to format numbers as currency by taking advantage of the Intl.NumberFormat()
constructor:
const formatCurrency = (amount, locale = 'en-US', currency = 'USD') => { return new Intl.NumberFormat(locale, { style: 'currency', currency: currency, }).format(amount);};
console.log(formatCurrency(369741564, 'en-NG', 'NGN')); // ₦369,741,564.00console.log(formatCurrency(369741564, 'it-EU', 'EUR')); // 369.741.564,00 €console.log(formatCurrency(369741564, 'en-GB', 'GBP')); // £369,741,564.00
Thank you for reading!