PHP Number Format Currency – How to Format Currencies in PHP
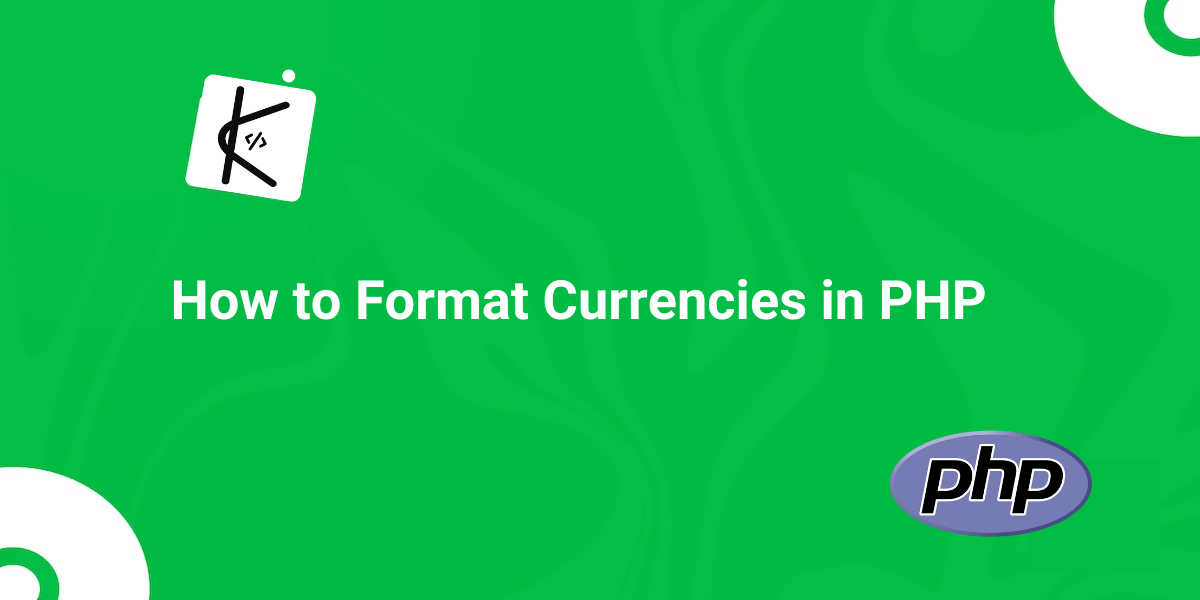
Written by Kolade Chris | Oct 5, 2024 | #PHP | 5 minutes Read
In eCommerce applications, financial reports, and other apps where currencies are integral, formatting strings or numbers as currency could be a regular task.
You can do this yourself and go through the stress of adapting the apps to several currencies, or take advantage of the built-in methods PHP offers for the same task.
In this article, you’ll learn how to manually format currencies and use the built-in PHP way of doing it more conveniently.
The Manual Approach: How to Format Currency in PHP with a Variable or Custom Function
You can use the number_format()
function as a quick workaround:
1$amount = 349023;2$formatMoney = number_format($amount, 2, '.', ',');3$currencySymbol = '$';4
5echo $currencySymbol . $formatMoney; // $349,023.00
For reusability, you could create a function that takes a number or string and converts it to a certain currency:
1function formatCurrency($amount)2{3 if (!is_numeric($amount)) {4 return 'Invalid amount entered!';5 }6
7 $amount = floatval($amount);8
9 $formattedAmount = number_format($amount, 2, '.', '');10
11 $parts = explode('.', $formattedAmount);12
13 $parts[0] = number_format($parts[0]);14
15 return '$' . implode('.', $parts);16}17
18echo formatCurrency('902.4'); // $902.4019echo "<br>";20echo formatCurrency(82.65); // $82.6521echo "<br>";22echo formatCurrency('ifdsa'); // Invalid amount entered!
The problem with this approach is that it handles one currency only.
To fix that, you can go ahead and make it accept a certain currency symbol with an extra parameter:
1function formatCurrency($amount, $currencySymbol)2{3 if (!is_numeric($amount)) {4 return 'Invalid amount entered!';5 }6
7 $amount = floatval($amount);8
9 $formattedAmount = number_format($amount, 2, '.', '');10
11 $parts = explode('.', $formattedAmount);12
13 $parts[0] = number_format($parts[0]);14
15 return $currencySymbol . implode('.', $parts);16}17
18echo formatCurrency('902.4', '$'); // $902.4019echo "<br>";20echo formatCurrency(82.65, '₦'); // ₦82.6521echo "<br>";22echo formatCurrency('ifdsa', '$'); // Invalid amount entered!
The problem with this manual approach is that it lacks locale-specific support and dynamic currency symbols, even though the number_format()
function accurately formats numbers.
The approach may also introduce precision issues and complexity with functions like explode
and implode
. In addition, handling invalid input can be cumbersome, and hardcoding currency symbols limit flexibility.
The solution is the NumberFormatter
class from the Intl
extension. Let’s look at how to use it.
How to Format Currencies with the PHP NumberFormatter
Class
Using the NumberFormatter
class from the Intl
extension to format currency is an upgrade over many manual solutions.
Here’s why:
- it helps you adapt the formatting to the conventions of different locales, such as decimal points and thousand separators
- it handles various currency symbols automatically based on the currency code you specify
- it lets you avoid precision issues that might happen with the use of
floatVal()
- it has cleaner code
Here are the steps to follow so you can use the NumberFormatter
class:
- set the locale and the amount:
1$dollarLocale = 'en_US';2$dollarAmount = 45237.89;
- create the
NumberFormatter
instance that takes in thelocale
and the kind of formatting you want, in this case, currency :
1$dollarFormatter = new NumberFormatter($dollarLocale, NumberFormatter::CURRENCY);
- call the
formatCurrency()
method on the instance. It takes the amount and currency code as parameters:
1$formattedDollar = $dollarFormatter->formatCurrency($dollarAmount, 'USD');
Here’s the full code:
1$dollarLocale = 'en_US';2$dollarAmount = 45237.89;3
4$dollarFormatter = new NumberFormatter($dollarLocale, NumberFormatter::CURRENCY);5$formattedDollar = $dollarFormatter->formatCurrency($dollarAmount, 'USD');6
7echo $formattedDollar; // $45,237.89
And here’s another example that formats the Naira:
1$nairaLocale = 'en_NG';2$nairaAmount = 45237.89;3
4$nairaFormatter = new NumberFormatter($nairaLocale, NumberFormatter::CURRENCY);5$formattedNaira = $nairaFormatter->formatCurrency($nairaAmount, 'NGN');6
7echo $formattedNaira; // ₦45,237.89
You can make the whole process a reusable code by creating a function that takes an amount
, currency
, and locale
as arguments:
1function formatCurrency($amount, $currency = 'USD', $locale = 'en_US')2{3 if (!is_numeric($amount)) {4 return 'Invalid amount entered!';5 }6
7 $formatter = new NumberFormatter($locale, NumberFormatter::CURRENCY);8
9 return $formatter->formatCurrency(floatval($amount), $currency);10}11
12echo formatCurrency('902.4', 'USD'); // $902.4013echo "<br>";14echo formatCurrency(8232.65, 'NGN', 'en_NG'); // ₦8,232.6515echo "<br>";16echo formatCurrency(5892309.93, 'EUR', 'it_IT'); // 5.892.309,93 €17echo "<br>";18echo formatCurrency('ifdsa', 'USD'); // Invalid amount entered!
Thank you for reading!