Next.js Layout Example – How to Add a Layout to your Next.js Website
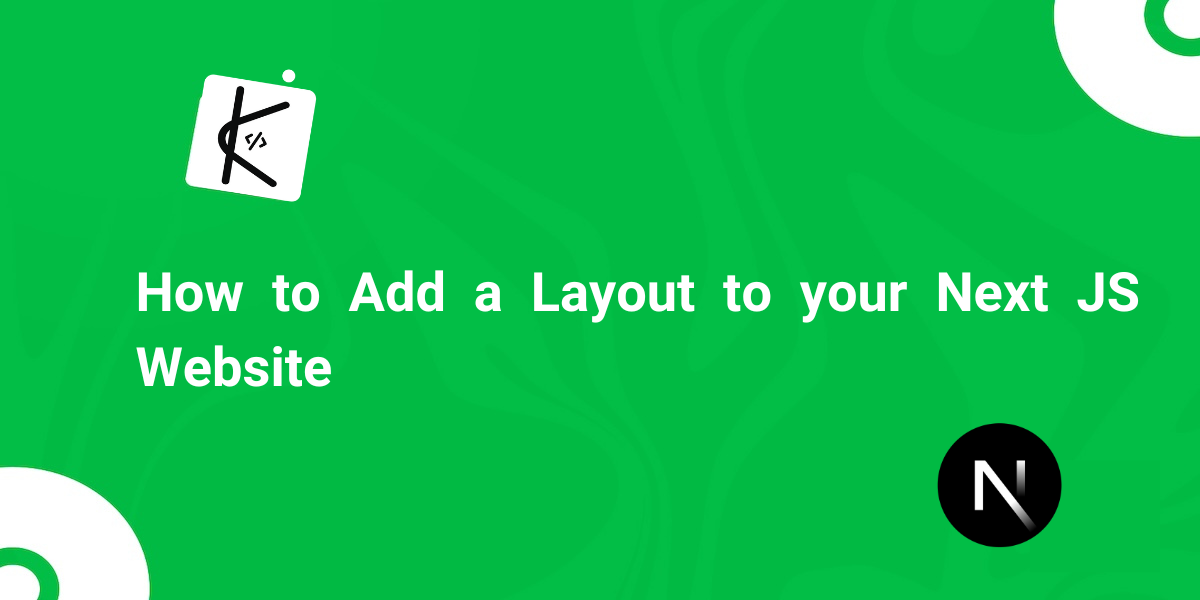
Written by Kolade Chris | Aug 24, 2024 | #Next.js #React | 5 minute Read
In Next.js, a layout file (AKA Next.js root layout) lets you have components that are common to all pages in one file. This could be headers, footers, and navigation menus. You then need to wrap the layout around all the pages of the website.
So, adding a layout to your React or Next.js website is a great way to give your website a uniform look across different pages.
In this article, you’ll learn how to add a layout to your Next.js website. We will be using the latest Next.js version, but you can apply the same approach to older versions. Also, it doesn’t look like the approach will change so much in the coming versions.
What you’ll Build
This is the preview of what you’ll build in this article:
As you can see, the parts of the website that are common to all pages did not change. This is the navbar and the footer.
How to Add a Layout to your Next.js Website
Step 1: Create a new Next.js project with the command below:
1npx create-next-app@latest # if you're using NPM2yarn create next-app # if you're using Yarn3pnpm create next-app # if you're using PNPM
The installer will take you through some prompts to select some options. Make sure you select the app router. That’s because this article will show you how to add a layout to your website using the Next.js app router.
Step 2: Create a layout.jsx
or layout.tsx
file if you’re using Typescript inside the app
directory.
Step 3: Create a MainLayout
functional component that takes in a children
prop and export it:
1const MainLayout = ({ children }) => {2 return <div>MainLayout</div>;3};4
5export default MainLayout;
Step 4: Import the components you want to have on all pages, like Navbar
and Footer:
1import '@/assets/styles/globals.css';2import Navbar from '@/components/Navbar';3import Footer from '@/components/Footer';4
5const MainLayout = ({ children }) => {6 return <div>MainLayout</div>;7};8
9export default MainLayout;
Step 5: Create the boilerplate tags you need, like html
, <body>
, <main>
and pass the children
prop into the <main>
tag:
1import '@/assets/styles/globals.css';2import Navbar from '@/components/Navbar';3import Footer from '@/components/Footer';4
5const MainLayout = ({ children }: { children: React.ReactNode }) => {6 return (7 <html>8 <body>9 <main>{children}</main>10 </body>11 </html>12 );13};14
15export default MainLayout;
Step 6: Use the Navbar
and Footer
components in the return statement:
1import '@/assets/styles/globals.css';2import Navbar from '@/components/Navbar';3import Footer from '@/components/Footer';4
5const MainLayout = ({ children }: { children: React.ReactNode }) => {6 return (7 <html>8 <body>9 <Navbar />10 <main>{children}</main>11 <Footer />12 </body>13 </html>14 );15};16
17export default MainLayout;
You can also add meta tags to all the pages the layout will have access to by exporting a metadata object this way:
1import '@/assets/styles/globals.css';2import Navbar from '@/components/Navbar';3import Footer from '@/components/Footer';4
5export const metadata = {6 title: 'Green World | Home of Nature Scenes',7 description: 'Find the coolest nature images and videos',8 keywords: 'nature animals trees fields',9};10
11const MainLayout = ({ children }: { children: React.ReactNode }) => {12 return (13 <html>14 <body>15 <Navbar />16 <main>{children}</main>17 <Footer />18 </body>19 </html>20 );21};22
23export default MainLayout;
That’s all you need to create a layout in Next.js and make it work! To better understand things, you can grab the code for this article from the project GitHub repo.
Join my Free Newsletter
Subscribe to my newsletter for coding tips, videos from reputable sources, articles from OG tech authors, and a ton of other goodies.
No BS. No fluff. Just pure software development goodies on a Sunday every week.
Wrapping Up
Are you wondering why you don’t need to import the layout.jsx
file into each page and wrap it around the content in it to make the layout work?
THat happens because Next.js looks out for the layout.jsx
or layout.tsx
file in any directory and automatically wraps it around the content of each page for you.
In the case of this article, the layout is located in the app
directory:
If you want the layout of a particular page to be different, you can override the root layout inside the app
directory by creating a layout.jsx
or layout.tsx
file inside the folder of that page.
Also, if you need multiple layouts, you can add a getlayout
property to your page, so you can return a React component for the layout. Read more about that in the Next.js docs.