How to Import a JSON File in JavaScript
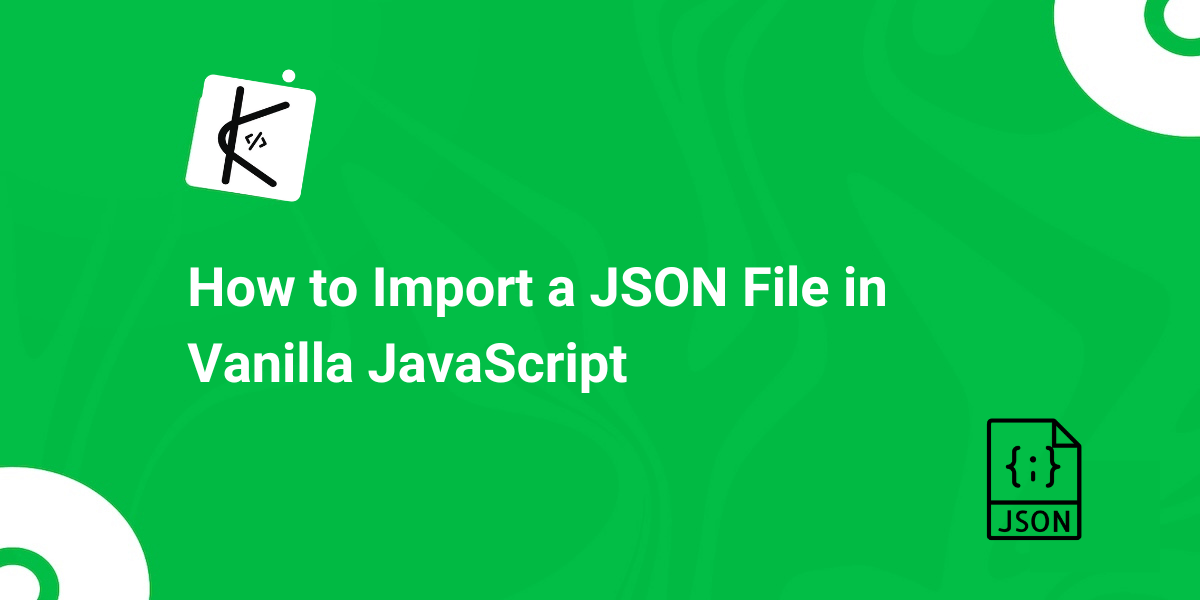
Written by Kolade Chris | Jun 15, 2024 | #JavaScript #WebDev | 3 minutes Read
JSON provides a lightweight and easy-to-parse data format for JavaScript projects. JavaScript’s native support for JSON enables efficient data handling and storage, making it easy to use in your codebases.
Importing JSON into a JavaScript file is not as straightforward as it is in frontend frameworks like React and Vue and a JavaScript runtime like Node JS.
Let’s examine the error that occurs when you try to import a JSON file into a vanilla JavaScript file directly and how you can fix it by correctly importing the JSON file. We’ll also examine a popular alternative to directly importing a JSON file in vanilla JavaScript.
The Problem: Directly Importing JSON Files in a Vanilla JavaScript File throws a SyntaxError
Error
In frontend frameworks like React and Vue, you can easily import any JSON file into your JS or JSX file using the import
and from
keywords:
1Import myJSONFile from `someFolder/someFile.json`
In a Node JS environment, you can import a JSON file the same way if you’ve specified type module
in your package.json
file:
1{2 "name": "my-project",3 "version": "1.0.0",4 "description": "Some description",5 "main": "server.js",6 "type": "module",7}
But if you do the same in a vanilla JavaScript codebase, you’ll get the error Uncaught SyntaxError: Cannot use import statement outside a module (at app.js:1:1)
:
Let’s look at how to correctly import a JSON file into a vanilla JavaScript file and subsequently fix that error.
Join my Free Newsletter
Subscribe to my newsletter for coding tips, videos from reputable sources, articles from OG tech authors, and a ton of other goodies.
No BS. No fluff. Just pure software development goodies on a Sunday every week.
How to Correctly Import a JSON File into a Vanilla JavaScript File
The process starts from your index.js
file, app.js
file, or any JS file in which you want to do the import.
Make sure you specify type module
in your script tag before you link the JavaScript file:
1<script type="module" src="app.js"></script>
Then inside the JavaScript file, import the JSON file using the import
and from
statements as usual, the add assert { type: 'json' }
to the end
1import JSONFileName from 'location/file.json' assert { type: 'json' };
In the case of the code I’m using, I have prepared a small JSON file showing a few African countries, their capitals, and currencies:
1{2 "countries": [3 {4 "name": "Nigeria",5 "capital": "Abuja",6 "currency": "Nigerian Naira"7 },8 {9 "name": "South Africa",10 "capital": "Pretoria",11 "currency": "South African Rand"12 },13 {14 "name": "Egypt",15 "capital": "Cairo",16 "currency": "Egyptian Pound"17 },18 {19 "name": "Morocco",20 "capital": "Rabat",21 "currency": "Moroccan Dirham"22 },23 {24 "name": "Tanzania",25 "capital": "Dodoma",26 "currency": "Tanzanian Shilling"27 }28 ]29}
Here’s how I did the import:
1import africaJson from './africa.json' assert { type: 'json' };
I successfully displayed the items by logging africaJSON.countries
:
1import africaJson from './africa.json' assert { type: 'json' };2
3console.log(africaJson.countries);
If you want to see other deeply nested attributes such as the name
, currency
, and capital
of the countries, you need to do some looping with forEach()
or map()
:
1import africaJson from './africa.json' assert { type: 'json' };2
3console.log(africaJson.countries);4
5const countryNames = africaJson.countries;6const countryCurrencies = africaJson.countries;7const countryCapitals = africaJson.countries;8
9countryNames.forEach((country) => {10 console.log(country.name);11});12
13console.log('\n');14
15countryCurrencies.forEach((country) => {16 console.log(`${country.name}'s currency is`, country.currency);17});18
19console.log('\n');20
21countryCapitals.forEach((country) => {22 console.log(`${country.name}'s capital is`, country.capital);23});
The Popular Alternative: How to Use the Fetch API
to Import a JSON File in Vanilla JavaScript
Most external APIs you use in JavaScript are JSON or arrays with objects. Then, you use the Fetch API
or axios
to display the data from that API response. You can do the same thing for a JSON file.
1fetch('./africa.json')2 .then((response) => response.json())3 .then((data) => {4 console.log(data);5 })6 .catch((error) => {7 console.error('Error Fetching JSON File:', error);8 });
Thank you for reading!